Are you tired of hardcoding passwords in your Spring Boot applications, exposing them to security vulnerabilities? Fortunately, there’s a solution – encryption! In this blog post, we’ll explore how to use Jasypt (Java Simplified Encryption) to encrypt your passwords for Spring Boot applications.
Jasypt(Java Simplified Encryption) is a tool that provides encryption support for property sources in Spring Boot Applications. This tool will help us to add basic encryption features with little effort.
How can we generate an Encrypted key?
There are 3 methods:
- The first one can be done by using an Online Tool on the following link
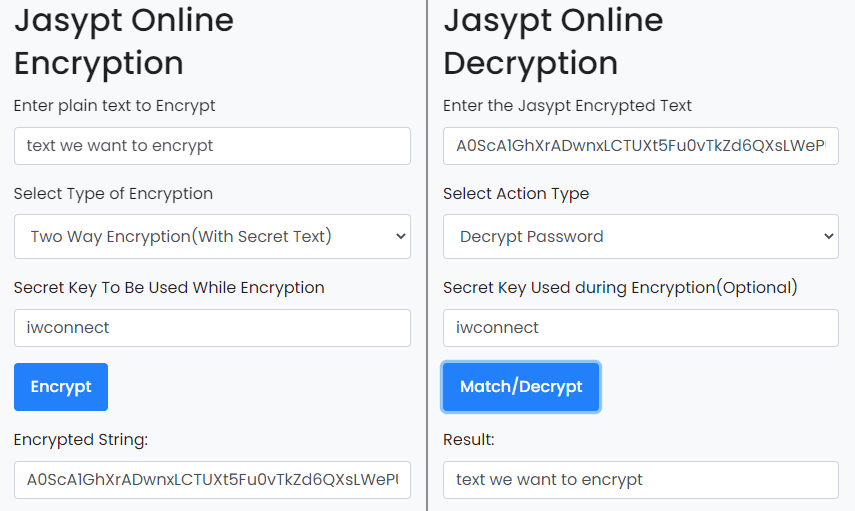
2. The second one is by using the jar Jasypt file
To generate an encrypted string using Jasypt jar file use the following command
java -cp jasypt-1.9.3.jar org.jasypt.intf.cli.JasyptPBEStringEncryptionCLI
input=<text-you-want-to-encrypt> password=<key-for-decrypting> algorithm=PBEWithMD5AndDES
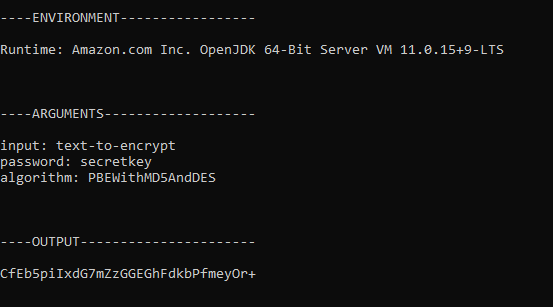
3. The third method is by using the Maven command
Use the following command for encrypting:
mvn jasypt:encrypt-value "-Djasypt.encryptor.password=password" "-Djasypt.plugin.value=test"
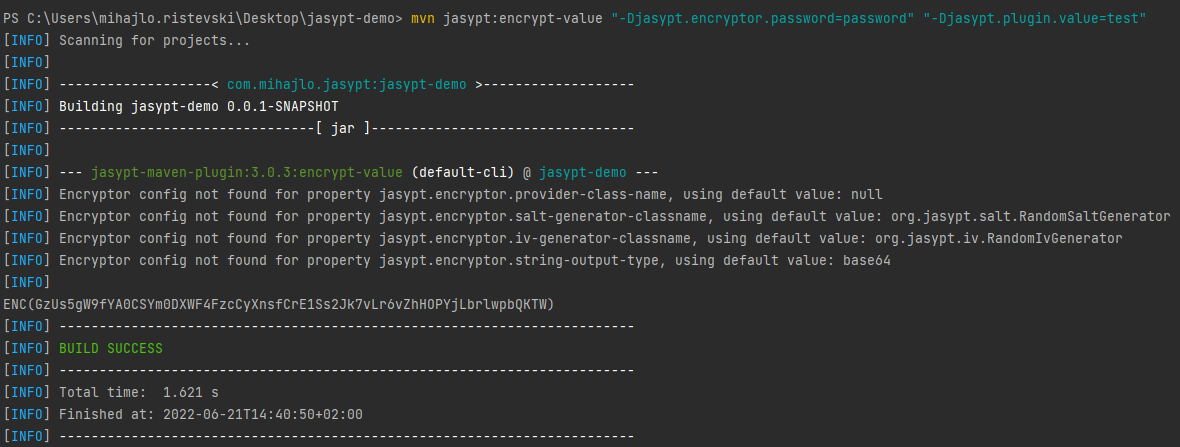
Use the following command for decrypting:
mvn jasypt:decrypt-value "-Djasypt.encryptor.password=password" "-Djasypt.plugin.value=<encrypted-value> "
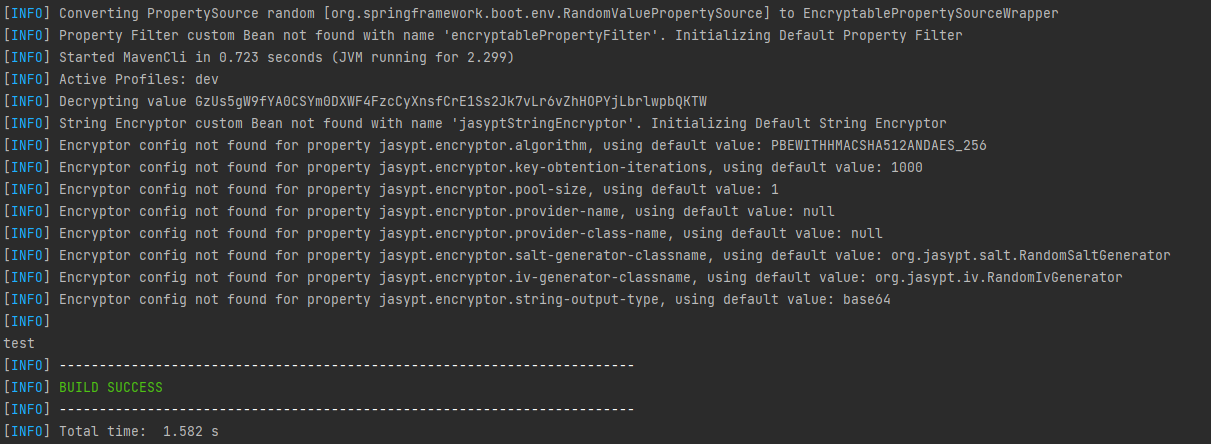
How to use Jasypt in Spring Boot
To integrate Jasypt with Spring Boot first you need to add the dependency in the pom.xml file
<dependency>
<groupId>com.github.ulisesbocchio</groupId>
<artifactId>jasypt-spring-boot-starter</artifactId>
<version>3.0.4</version>
</dependency>
The next thing is to add the @EnableEncryptableProperties annotation in your Spring Boot Application Main Configuration class. In this blog post, we will implement the encrypted value in an Environment Variable and configure the Spring Boot Application to read it from there. (We are using the h2 database in our example).
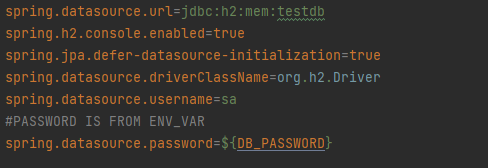
The next thing we have to do is to open our Environment Variables and add the DB_PASSWORD variable and the value for it, which is encrypted using the Jasypt jar file. To Access the Environment Variables:
- Right-click on My Computer
- Select Properties
- Select Advanced System Settings
- Select Environment Variables
To enter a new Environment Variable click on the button New and a window like the one below will pop out where you have to insert a Variable name and Variable value. With this method, you will add the value permanently to your Environment Variables.
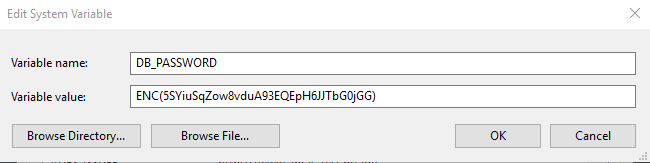
The other way to add an Environment Variable is by using the Command Line. This method can set the variable for the current CMD Session. Only when we restart the session we have to set the variable again. The command for that is the following:
set varname=value
Example
set DB_PASSWORD=ENC(<encrypted-value-from-jasypt>)
In order for Jasypt to recognize your encrypted values, you must follow a specific convention and format, which you can see below. This will ensure that Jasypt can properly decrypt your encrypted passwords in your Spring Boot application.
ENC(encrypted key) -> ENC(scEjemHosjc/hjA8saT7Y6uC65bs0swg)
Secret key needs to be passed to decrypt at runtime:
Make the Jasypt aware of the secret key which you have used to form the encrypted value.
The following are different methods to pass the secret key:
1. Pass it as a property in the config file. Run the project as usual and the decryption would happen.
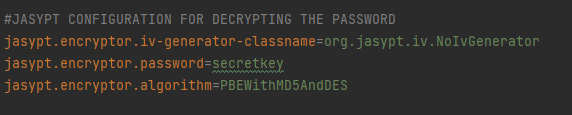
2. Run the project with the following command:
java -Xms256m -Xmx512m -Djasypt.encryptor.password=<secret-key> -Djasypt.encryptor.algorithm=PBEWithMD5AndDES -jar jasypt-demo-0.0.1-SNAPSHOT.jar
3. Export Jasypt Encryption Password as an Environment Variable:
JASYPT_ENCRYPTOR_PASSWORD=secretkey
Git Hub Repository: jasypt-demo
Prerequisites
- Java JDK 17
Installation
Clone the Github Repository or download the zip file
- Get the project from GIT “git clone https://github.com/mihajloristevski25/jasypt-demo.git”
- Set datasource url of your H2 DB application.properties -> spring.datasource.url
- Set H2 user value into application.properties -> spring.datasource.username
Usage:
- Open the root directory inside of it is a jar file called jasypt-1.9.3.jar you will need this file to encrypt the value.
- Use the folowing command to generate the encrypted value
java -cp jasypt-1.9.3.jar org.jasypt.intf.cli.JasyptPBEStringEncryptionCLI input=<value-to-encrypt> password=<secret-key> algorithm=PBEWithMD5AndDES
- Set the password for the DB in an Environment variable
- Package the app in a jar using maven command
mvn clean package -DskipTests
- In the target folder in root directory is the jar
- To start the Application use the folowing command
java -Xms256m -Xmx512m -Djasypt.encryptor.password=<secret-key> -Djasypt.encryptor.algorithm=PBEWithMD5AndDES -jar jasypt-demo-0.0.1-SNAPSHOT.jar
Open the browser and on http://localhost:8080/h2-console and enter your credentials and log in the database.