The process of AES encryption and decryption in SnapLogic is implemented in the AES Encrypt and AES Decrypt snaps available in the standard Binary Snap Pack. Snaps support multiple cipher modes and PKCS5 and ISO10126 encryption paddings. This article describes a solution for you as an integration architect for AES CBC PKCS7 encryption and decryption with Script snap and Bouncy Castle library. Similarly, SnapLogic can be further extended with other cryptography modes as implemented in the Bouncy Castle Java library.
Background
AES or Advanced Encryption Standard is a symmetric encryption algorithm. Symmetric cryptography algorithms use the same key in encryption and decryption processes. The CBC part which stands for Cypher-Block-Chaining is a mode of operation where the input string is divided into multiple parts of the block size. Considering the variable length of the input a padding mechanism is used. In this case, PKCS7 denotes the padding algorithm. The PKCS7 padding allows block sizes of up to 255 bytes compared to PKCS5 padding which is only 8 bytes long. Padding works by appending bytes in order to make the final block of data the same size as the block size.
Problem
In SnapLogic the standard AES Encrypt and AES Decrypt snap do not support PKCS7 padding out of the box. However, considering that the existing cryptography snaps are built on the Bouncy Castle library we could potentially use the same library with the Script snap to solve our problem. If we look at the SnapLogic list of provided platform libraries we can find a reference to the Bouncy Castle library version 1.49. This means that Bouncy Castle cryptography objects can be instantiated in Script Snap without additional jar files.
Nashorn JavaScript engine
Nashorn Javascript Engine is a technology developed by Oracle and part of Java SE 8. This engine allows dynamic execution of JavaScript code in the JVM. The Nashorn Javascript Engine competes with other standalone engines like Google V8. SnapLogic Script snap executes a Javascript, Python, or Ruby script using this JVM Script engine mechanism. Scripts can be executed locally for example during the development process by using the jjs tool. JJS is an acronym for Java JavaScript. JJS tool is part of Java SE 8 and allows interpretation of commands or script files.
Solution
The solution will be implemented in JavaScript notation. The following instructions saved in a .js can be executed locally by using jjs. The -cp is a class path option.
jjs -cp bcprov-jdk15on-165.jar sample.js
First, import the required packages:
importPackage(java.util);
importPackage(java.io.UnsupportedEncodingException);
importPackage(java.security);
importPackage(javax.crypto);
importPackage(java.nio);
Next define key, initialization vector, and encrypted value. Values are Base64 encoded.
var value_to_decode = new java.lang.String();
value_to_decode = “DICDmOLVSQKoxtH2Xz6v3A==”;
var KEY_AES = new java.lang.String();
KEY_AES = “FUi0iidyYDpBl+WTqB8EZJAT+cjkIkH8nuXNMGRoQvk=”;
var IV = new java.lang.String();
IV = “fspJSRCbLtfQaVtKDaOJEg==”;
var key_array = Java.type(“byte[]”);
var iv_array = Java.type(“byte[]”);
var encrypted_array = Java.type(“byte[]”);
key_array = java.util.Base64.getDecoder().decode(KEY_AES);
iv_array = java.util.Base64.getDecoder().decode(IV);
encrypted_array = java.util.Base64.getDecoder().decode(value_to_decode);
Next, register the Bouncy Castle security provider
Security.addProvider(new org.bouncycastle.jce.provider.BouncyCastleProvider());
Next prepare key specifications, parameters, and cipher
var secretKeySpec = new javax.crypto.spec.SecretKeySpec(key_array, “AES”);
var parameters = java.security.AlgorithmParameters.getInstance(“AES”);
parameters.init(new javax.crypto.spec.IvParameterSpec(iv_array));
var cipher = Java.type(“javax.crypto.Cipher”);
cipher = cipher.getInstance(“AES/CBC/PKCS7Padding”, “BC”);
cipher.init(Cipher.DECRYPT_MODE, secretKeySpec, parameters);
Finally, execute the decryption process
var decoded_array = Java.type(“byte[]”);
decoded_array = cipher.doFinal(encrypted_array);
var decoded = new java.lang.String(decoded_array);
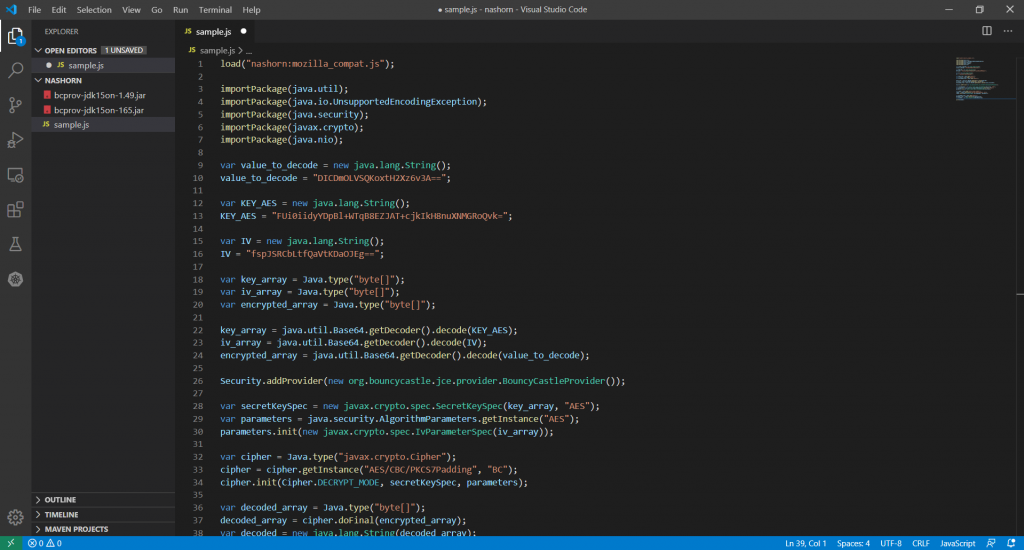
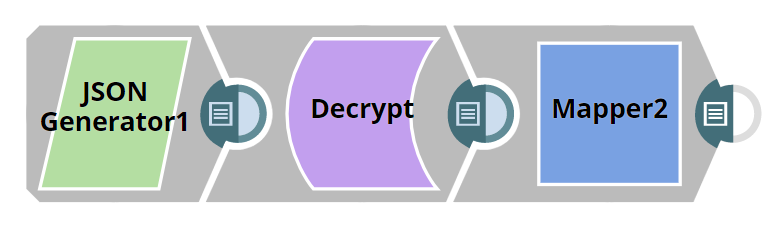
Final considerations
The encryption key along with the initialization vector and encrypted data can be part of the input document, and decrypted data can be stored in a new field as part of the output document. The encryption material should be well protected at rest. This imposes limited permission only to authorized users and is stored in encrypted form. One possible solution could be using PGP encryption and decryption snaps with a preconfigured account for this purpose.