Introduction
Cypress is a popular end-to-end testing framework that is commonly used for testing web applications. It provides a robust set of tools that allows developers and testers to write and execute automated tests, ensuring the quality of the software being developed. In addition to testing the user interface of an application, Cypress also provides support for testing APIs.
API testing is the practice of testing the interfaces of software components to ensure that they function correctly and meet the requirements of the application. This type of testing is often used to validate the correctness of data being exchanged between different software components. API testing can be challenging, especially when dealing with complex software systems. However, Cypress provides a simple and intuitive way to test APIs, allowing testers to verify the behavior of backend services in addition to frontend user interfaces.
API testing with Cypress is a powerful tool for developers and testers to ensure the functionality of their APIs. Cypress provides an intuitive and easy-to-use interface for writing API tests, with several functionalities that make testing a breeze. These functionalities include:
- Making HTTP requests: Cypress provides an easy way to make HTTP requests to your API, allowing you to test its functionality.
- Assertions: Cypress provides a suite of assertions that allow you to ensure that your API is returning the expected responses. You can use these assertions to check the response status, the response body, or any other aspect of the API response.
- Mocking: Cypress allows you to mock API responses, enabling you to test the behavior of your application
- Spying: Cypress enables you to spy on network requests made by your application, allowing you to validate the API response and ensure that your application is working as intended.
- Chaining requests: Cypress allows you to chain requests together, enabling you to test complex workflows that involve multiple API calls.
Overall, API testing with Cypress is a powerful and efficient way to test your APIs and ensure that they are working as intended. In the following blog post, we are going to use Cypress for API testing.
Cypress configuration for UI and API testing
Cypress is an open source JavaScript end-to-end testing framework for web applications. It provides a quick, reliable, and simple way to test your application’s user interface and behavior. Cypress is designed to work with React, Angular, Vue.js, and other modern front-end frameworks.
Cypress has a unique architecture that allows you to write tests that closely resemble how users interact with your application. This makes it easier to write tests that accurately reflect your application’s behavior and catch bugs early in the development process.
Cypress provides powerful features for testing web applications, e.g.
- Automatic wait for page elements to load
- Real-time reloading of tests as you make changes
- Screenshots and animated videos of failed tests
- A simple and intuitive API for writing tests
- Ability to test and debug using Chrome DevTools
Overall, Cypress is a powerful testing framework that can help you optimize your web applications and catch bugs before they reach production. Most testing tools (like Selenium) operate by running outside of the browser and executing remote commands across the network Cypress is the exact opposite, Cypress is executed in the same run loop as your application.
Behind Cypress is a Node server process. Cypress and the Node process constantly communicate, synchronize, and perform tasks on behalf of each other.
Installing Cypress
System Requirements
Cypress is a desktop application that is installed on your computer, and supports these operating systems:
- MacOS 10.9 and above (Intel or Apple Silicon 64-bit (x64 or arm64))
- Linux Ubuntu 12.04 and above, Fedora 21 and Debian 8 (x86_64 or Arm 64-bit (x64 or arm64)
- Windows 7 and above (64-bit only)
Install Visual Studio Code that can be found on the official site and install Node.js from the official site.
Open Visual Studio Code and Install Cypress via npm.

We are using npm to install Cypress, we support:
- Node.js 14.x
- Node.js 16.x
- Node.js 18.x and above
Now you can open Cypress from your project root one of the following ways:

Adding npm scripts
Into the package.json and under the scripts file we will set up framework scripts. We basically need three of them, at least one for opening cypress, one for an ink test in headless mode and one for updating the snapshots, and one for browser selection. “cy:open“: “cypress open“, “cy:run“: “cypress run“, “cy:run:edge“: “cypress run –browser edge“, we will also later set up another one for cypress dashboard.
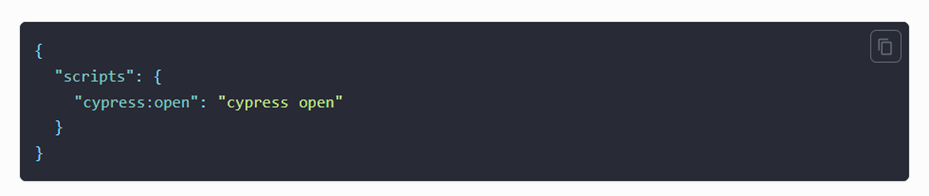

Now you can invoke the command from your project root like so:


After configurating the package.json file with the dependencies and framework scripts we will now set up the framework structure.
Set up Page Object model structure
Page Object Model (POM)is a design pattern, popularly used in test automation that creates Object Repository for web UI elements. The advantage of the model is that it reduces code duplication and improves test maintenance. We used this model and divide the test, locators & data and functions.
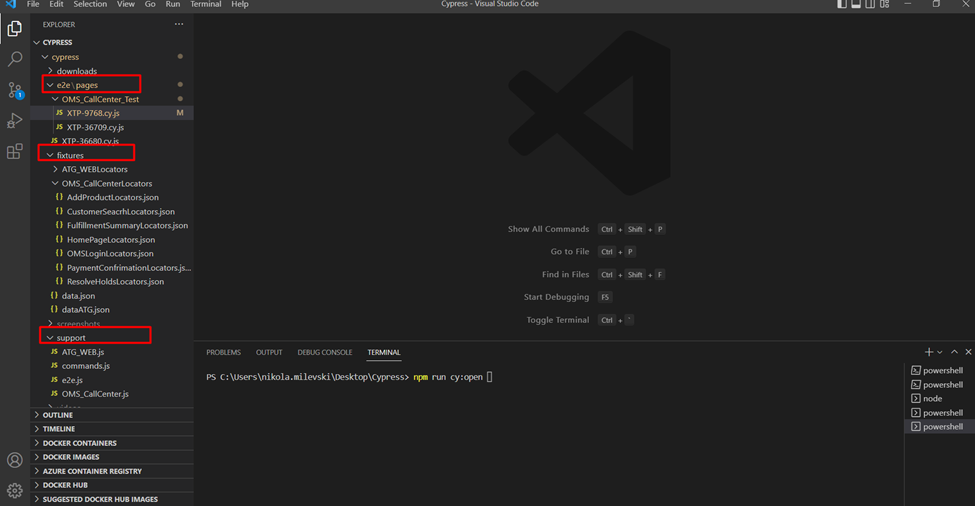
Use of the API request in Cypress
End-to-end test with Cypress
In this blog we will cover one test between two systems where we will present the usage of API request. First we will describe the end to end test from one external system and one internal system.
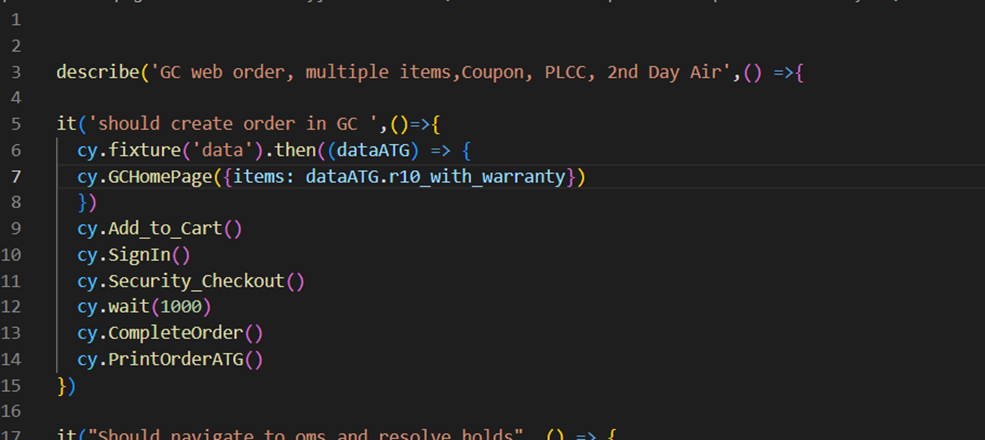
In the screenshot above, the user navigates to the external WEB system and creates order with item and warranty. The order number from the web system is stored into variable that will be sent through the API and will be used into the internal system.
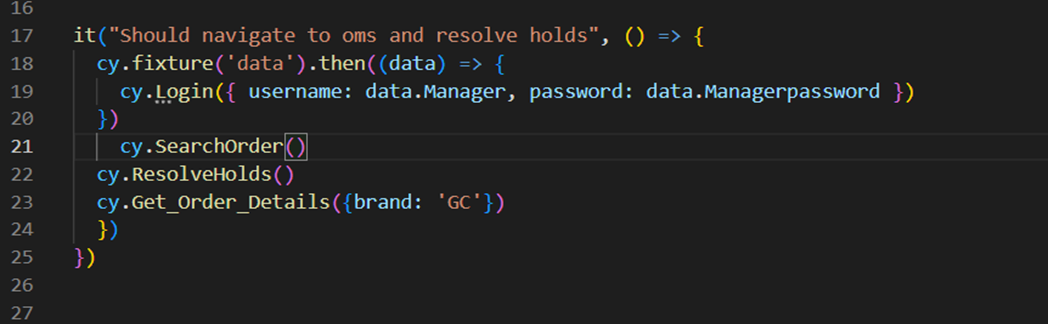
In the second screenshot above, the user goes on to navigate to the internal IBM system to search for the order that was processed by the API. We will delve into the details of the API request in the next chapter.
API Request
API stands for Application Programming Interface. It is a set of rules and protocols that allows different software applications to communicate with each other. APIs define how different software components should interact, specifying the types of requests and responses that can be made.
To make an API request using Cypress, we can use the ‘cy.request()’ command. After making the request, we can use the ‘then()’ method to handle the response and for asserting the response we can use the ‘expect()’ assertion. For our project we are using custom command In Cypress for retrieving order details from a specific API endpoint.
Cypress.Commands.add('Get_Order_Details', function (Order)
{
cy.request('https://Automation.practice.com/InteropHttpServlet?YFTEnvironment.progId=SterlingHttpTester&InteropApiName=processOrderPayments&IsFlow=N&ServiceName=&ApiName=processOrderPayments&YFTEnvironment.userId=test.testovski$TV&YFTEnvironment.password=testing%8554F%52&YFTEnvironment.version=&YFTEnvironment.locale=&InteropApiData=%3COrder+OrderNo%3D%22' + this.orderNo + '%22+DocumentType%3D%220001%22+EnterpriseCode%3D%22'%22%2F%3E')
.then((response) => {
expect(response.status).to.eq(200)
let strResponse = response.body
const orderHeaderKey = strResponse.match('OrderHeaderKey="(.+?)"')[1]
cy.log(orderHeaderKey)
cy.request('https://Automation.practice.com/InteropHttpServlet?YFTEnvironment.progId=SterlingHttpTester&InteropApiName=scheduleOrder&IsFlow=N&ServiceName=&ApiName=scheduleOrder&YFTEnvironment.userId=test.testovski$TV&YFTEnvironment.password=testing%8554F%52&YFTEnvironment.version=&YFTEnvironment.locale=&InteropApiData=%3CScheduleOrder%20CheckInventory%3D%22Y%22%20IgnoreMinNotificationTime%3D%22N%22%20OrderHeaderKey%3D%22' + orderHeaderKey + '%22%20%2F%3E')
cy.request('https://Automation.practice.com/InteropHttpServlet?YFTEnvironment.progId=SterlingHttpTester&InteropApiName=releaseOrder&IsFlow=N&ServiceName=&ApiName=releaseOrder&YFTEnvironment.userId=test.testovski$TV&YFTEnvironment.password=testing%8554F%52&YFTEnvironment.version=&YFTEnvironment.locale=&InteropApiData=%3COrder+OrderHeaderKey%3D%22' + orderHeaderKey + '%22%2F%3E')
})
})
- The custom command ‘Get_Order_Details’ takes an Order object as a parameter.
- The code uses the ‘cy.request()’ function to make HTTP requests to various API endpoints, the URLs in the requests include specific query parameters and data required by the API, after each request the code performs different actions based on the response.
- The first ‘cy.request()’ makes a request to retrieve order details using the processOrderPayments API. It expects a successful response with a status code of 200, then extracts the orderHeaderKey from the response body using a regular expression match and logs it.
- The next ‘cy.request()’ is for scheduling the order using the scheduleOrder API. It includes the orderHeaderKey obtained from the previous response.
- The variable this.orderNo is the order number that we stored from the WEB external system and we are calling it in the API for getting the order details.
- Finally the last ‘cy.request()’ releases the order using the releaseOrder API. The ‘orderHeaderKey’ obtained from the previous response is included in the request URL.
- The final ‘cy.request()’ is made to the releaseOrder API to release the order. The orderHeaderKey is included in the request URL.
- The code uses the ‘then()’ function to handle the response of each ‘cy.request()’ call. In our case it checks if the status code of the first request is 200 using expect(response.status).to.eq(200) and it also extracts the orderHeaderKey from the response body and logs it. It’s important to note that the URLs in the code are specific to a particular API endpoint.
Conclusion
In conclusion, Cypress is a powerful and user-friendly open-source framework for conducting end-to-end testing of web applications. With its intuitive JavaScript API, time-traveling feature, automatic waiting, and real-time reloading capabilities, Cypress offers a seamless testing experience.
The framework’s emphasis on simplicity, along with its robust debugging options, makes it a popular choice for automating tests and ensuring the quality of web applications, but it also has some drawbacks that you should be aware of:
- Limited mobile device testing: Cypress has limited support for mobile device testing. While it can be used to test responsive web applications, it does not provide extensive capabilities for mobile-specific testing scenarios.
- Lack of parallel execution: Cypress executes tests in a single browser instance, which can impact execution speed and scalability for large test suites. Parallel execution across multiple browser instances is not directly supported
- Single-domain limitation: Cypress operates within the same origin policy, which restricts cross-domain interactions. This can be a limitation when testing applications that make requests to multiple domains.
- No support for non-UI testing: Cypress is primarily focused on end-to-end testing of web applications with a user interface. If you need to perform non-UI testing, such as unit testing or API testing, you may need to use additional tools or frameworks alongside Cypress.
Authors:
Nikola Milevski | Hristijan Ivanovski