What is Clean Architecture?
Clean architecture is an architectural pattern that structures an application into distinct layers based on their responsibilities. The main idea behind clean architecture is the separation of concerns, ensuring that business logic is independent of frameworks, databases, and external systems. This makes applications easier to maintain, test, and extend over time.
Layers of Clean Architecture
Clean architecture typically consists of the following layers:
- Domain layer– contains core business rules and domain models. This layer should be independent of any external dependencies.
- Application layer – contains application-specific business logic, such as workflows and orchestrating interactions between entities and external systems.
- Infrastructure layer – bridges the gap between the core application logic and external frameworks, databases, or APIs.
- Presentation layer – includes web frameworks, UI components
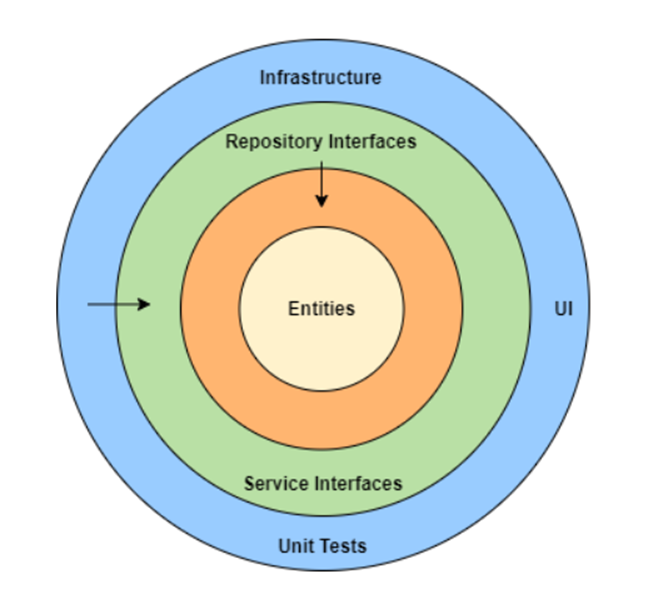
Picture 1 – Clean architecture diagram
Domain layer VS Application layer
One of the biggest sources of confusion in clean architecture is distinguishing between the domain layer and the application layer. Here’s a clear breakdown of their roles:
Domain Layer contains pure business rules that remain consistent regardless of the application’s external dependencies.
Example: A rule that ensures an order cannot be placed if an item is out of stock.
Application Layer implements use cases and workflows, orchestrating how different domain entities interact.
Example: Processing an order by coordinating inventory checks, payment processing, and notifications.
As Robert C. Martin explains in Clean Architecture,
“If a rule would exist even if you changed the technology stack (e.g., switched from .NET to Java), it belongs in the domain layer. If a rule is about how things are executed within the system (e.g., invoking an API or a database operation), it belongs in the application layer.”
Technology changes on Azure messaging services example
One of the biggest advantages of clean architecture is its ability to decouple business logic from technology choices. Since dependencies are inverted, replacing a database, UI framework, or messaging service does not impact the core business rules. This makes it easier to adapt to new technologies over time.
Imagine you initially designed your system using Azure Service Bus for handling event-driven messaging. Over time, as your application’s needs evolve, you realize that Azure Event Hub is a better fit due to its ability to handle high-throughput, real-time event streaming.
In a traditional tightly coupled architecture, this transition would be painful—you’d need to modify large portions of your codebase, refactor business logic, and potentially rewrite entire workflows. However, with clean architecture, the impact is minimal because the business logic remains untouched.
Let’s examine this change in an Order Processing System, where a messaging service is used whenever a product’s inventory needs to be updated. Here’s how the transition might look in practice:
- Define an interface – the application layer should not care whether we are using Azure Service Bus or Event Hub.
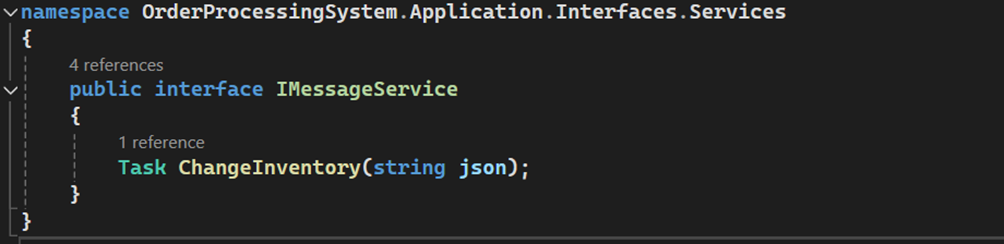
Picture 2 – IMessageService interface
- Implement the new service – create a new implementation of IMessageService that interacts with Azure Event Hub instead of Azure Service Bus.
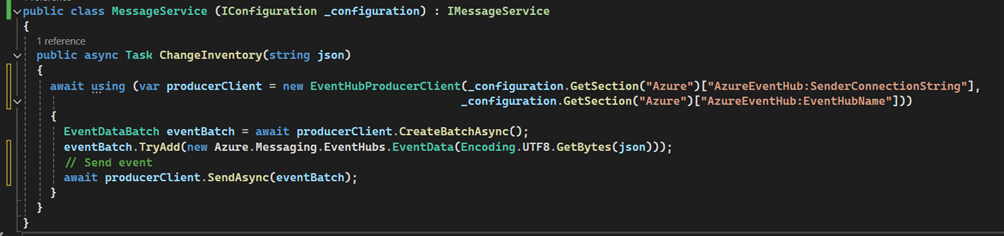
Picture 3 – Message service
- Swap out the old implementation – modify the dependency injection (DI) configuration to register the new Azure Event Hub implementation while keeping the rest of your application unchanged.
- Test and deploy – validate that messages are being processed correctly and seamlessly transition to the new service.
By following this approach, you can switch technologies in a matter of hours or days instead of undergoing massive refactoring. Clean architecture ensures that your core business rules remain independent of the infrastructure, making future technology changes smooth and risk-free.
Is Clean Architecture Always the Right Choice?
Clean architecture is especially beneficial for large-scale systems that require long-term maintainability and flexibility. However, for smaller or short-term projects, it can introduce unnecessary complexity and overhead.
While clean architecture offers many advantages, it also comes with challenges. One of the main difficulties is that it requires more time and effort to set up properly. Additionally, if the development team consists of multiple developers, it can become chaotic, especially if at least one team member is not fully familiar with clean architecture principles.
Another challenge when implementing clean architecture is applying its principles to existing software projects. This can be particularly difficult if the project is large and complex, as it may require significant restructuring of the codebase. In some cases, existing code may even need to be rewritten to fully conform to clean architecture guidelines.
Summary
Clean architecture provides a structured approach to software design by ensuring separation of concerns, maintainability, and flexibility. One of its key advantages is the ability to adapt to changing technologies without affecting core business logic, but requires careful consideration as it can introduce complexity and overhead if not properly implemented. By understanding how clean architecture handles such transitions, developers can future-proof their applications, ensuring adaptability to new technologies with minimal disruption.